In today’s fast-paced, data-driven world, efficiency is everything. As datasets continue to grow and the demand for rapid insights increases, data scientists and analysts need smarter tools to keep up. That’s where Data Analysis & Machine Learning with ChatGPT comes into play. ChatGPT, an AI-powered language model, has emerged as a powerful ally in the Python ecosystem—helping users streamline data workflows, build machine learning (ML) pipelines, and interpret complex results with ease.
This comprehensive guide explores the full potential of Data Analysis & Machine Learning with ChatGPT, showing you how to leverage the tool for everything from writing data analysis scripts using Pandas, NumPy, and Matplotlib, to constructing and explaining ML models with scikit-learn, and even optimizing your code for performance. By the end, you’ll understand how AI-assisted coding can transform your Python-based data science projects and supercharge your productivity.
Table of Contents
Introduction to ChatGPT for Data Analysis and Machine Learning
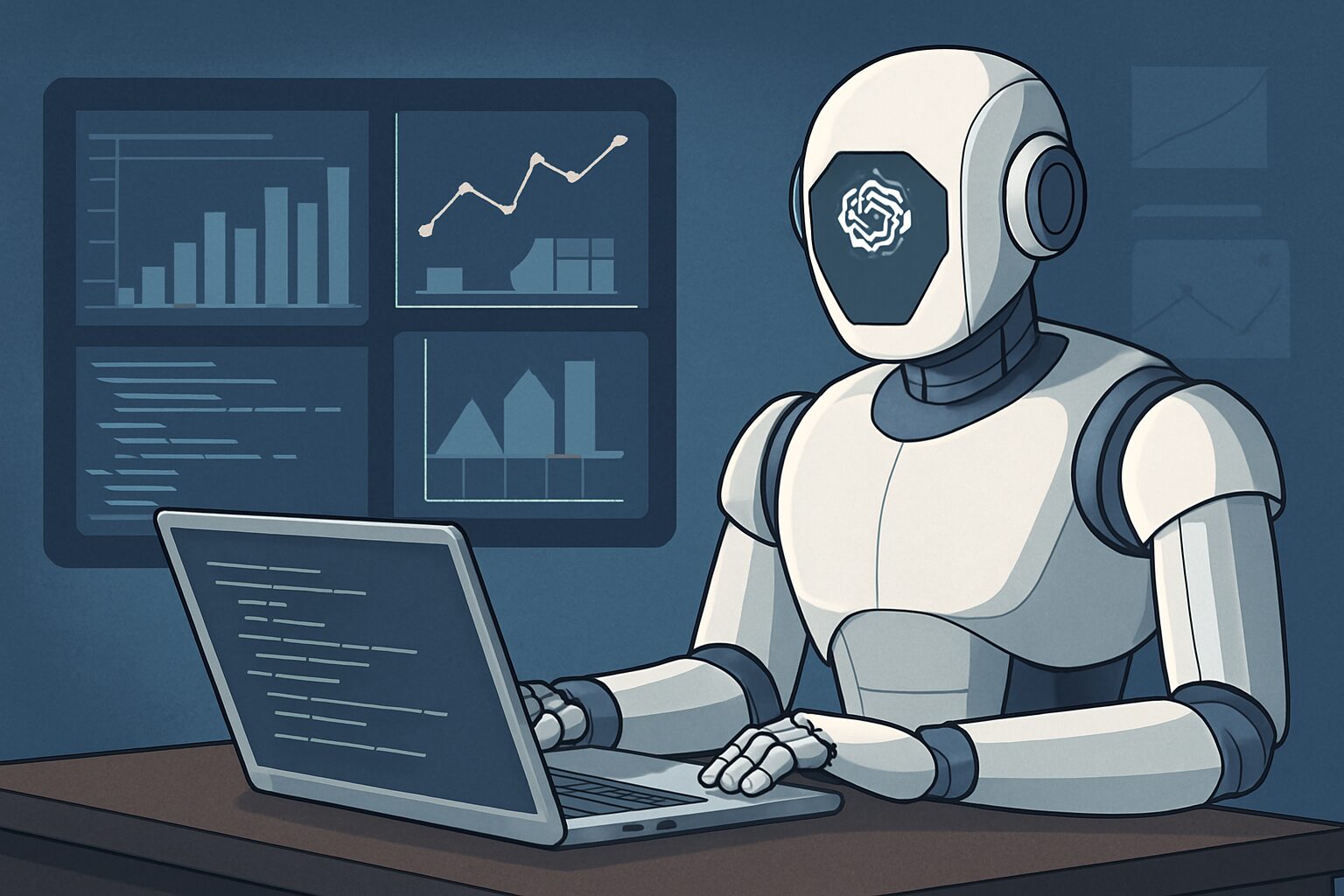
What Is ChatGPT?
ChatGPT is an AI-powered large language model developed by OpenAI. It’s designed to understand prompts and generate human-like text responses, making it incredibly useful for tasks that involve code generation, explanations, and creative problem-solving. For data analysis and machine learning practitioners, ChatGPT can serve as a coding assistant that writes and reviews Python scripts, explains algorithms, and even suggests best practices.
Why Use ChatGPT for Data Analysis & ML?
- Accelerated Development: ChatGPT can quickly draft boilerplate code for data loading, cleaning, visualization, and modeling.
- Reduced Errors: By suggesting syntax and logic, ChatGPT helps minimize common coding mistakes.
- Learning Aid: Newcomers to Python or data science can ask ChatGPT questions and get immediate, context-relevant answers.
- Time Savings: Routine tasks such as repetitive data cleaning steps or template generation can be offloaded, allowing you to focus on insights.
Key Benefits & Common Use Cases
- Exploratory Data Analysis (EDA): Prompt ChatGPT for scripts that summarize data sets, generate descriptive statistics, and produce plots.
- Machine Learning Modeling: Get end-to-end code for ML pipelines—from data splitting to model tuning.
- Code Explanation and Debugging: Paste in error messages or code snippets to get troubleshooting help.
- Optimization and Best Practices: Ask ChatGPT how to optimize your code, leverage vectorization, or select the right hyperparameters.
Setting Up Your Python Environment
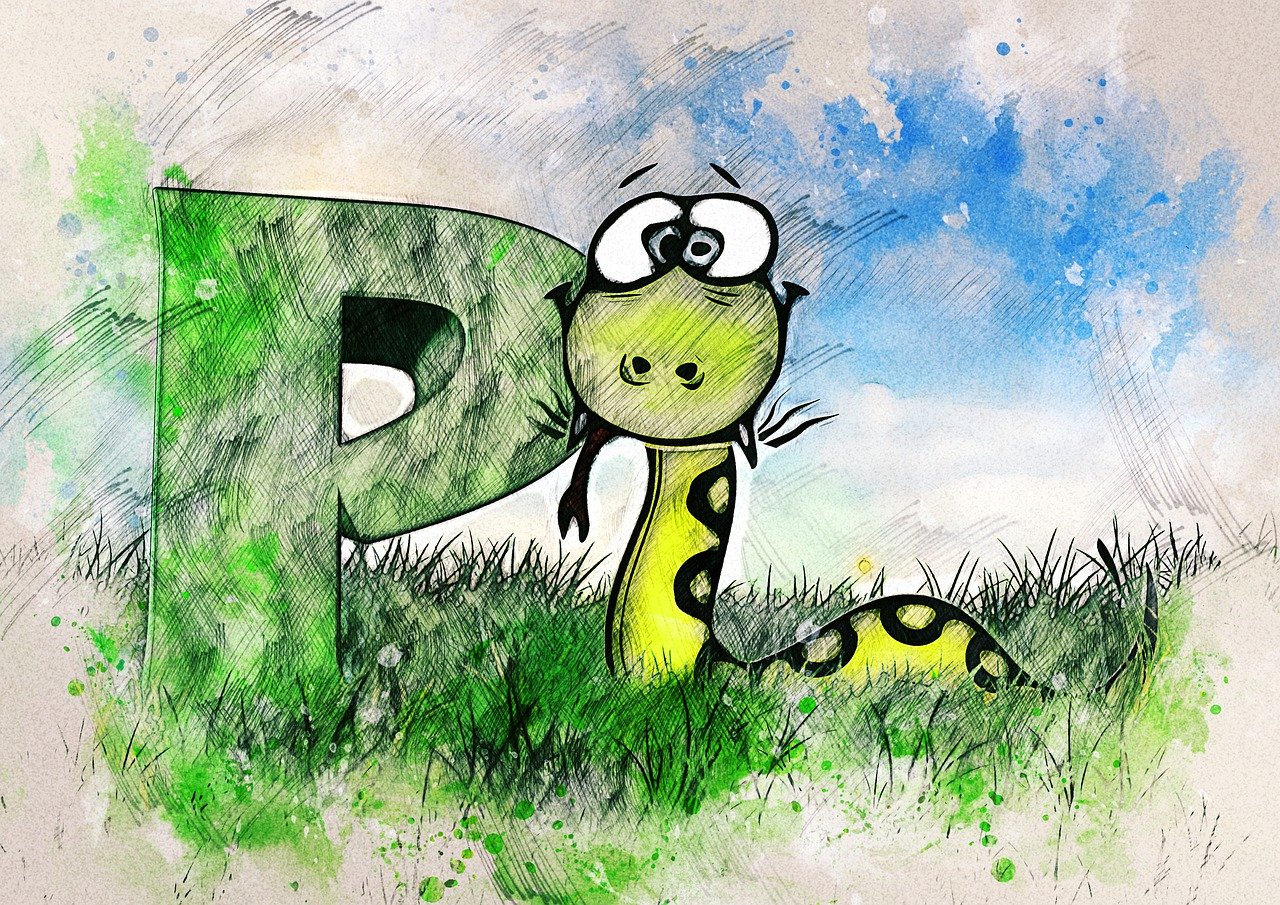
While ChatGPT can assist you in writing code, you’ll still need a proper development environment to run and manage your data science projects.
Installing Essential Libraries
To get started with data analysis and machine learning in Python, you’ll need to install the following core libraries:
- Pandas: For data manipulation and wrangling.
- NumPy: For efficient numerical computations.
- Matplotlib: For basic visualization.
- scikit-learn: For building ML models and pipelines.
- Jupyter Notebook or JupyterLab (optional but highly recommended for interactive development).
An example command to install these libraries would be:
pip install pandas numpy matplotlib scikit-learn jupyter
IDE Choices and Integrations
- Jupyter Notebook/Lab: Ideal for exploratory data analysis; you can integrate ChatGPT suggestions by copy-pasting snippets.
- Visual Studio Code (VSCode): Has extensive support for Python, code formatting, and version control. Plugins exist that can integrate ChatGPT directly or allow copy-pasting from ChatGPT.
- PyCharm: A robust Python IDE that supports debugging, virtual environments, and version control. While no official ChatGPT plugin exists, you can copy-paste ChatGPT snippets into the editor.
Best Practices for Version Control
No matter your IDE, it is vital to track changes to your code. Use Git for version control and a platform like GitHub or GitLab for cloud-based backups and collaboration:
- Create a repository for each project.
- Commit changes frequently with meaningful messages.
- Use branches for feature development and merge them into main after testing.
Generating Data Analysis Scripts with ChatGPT
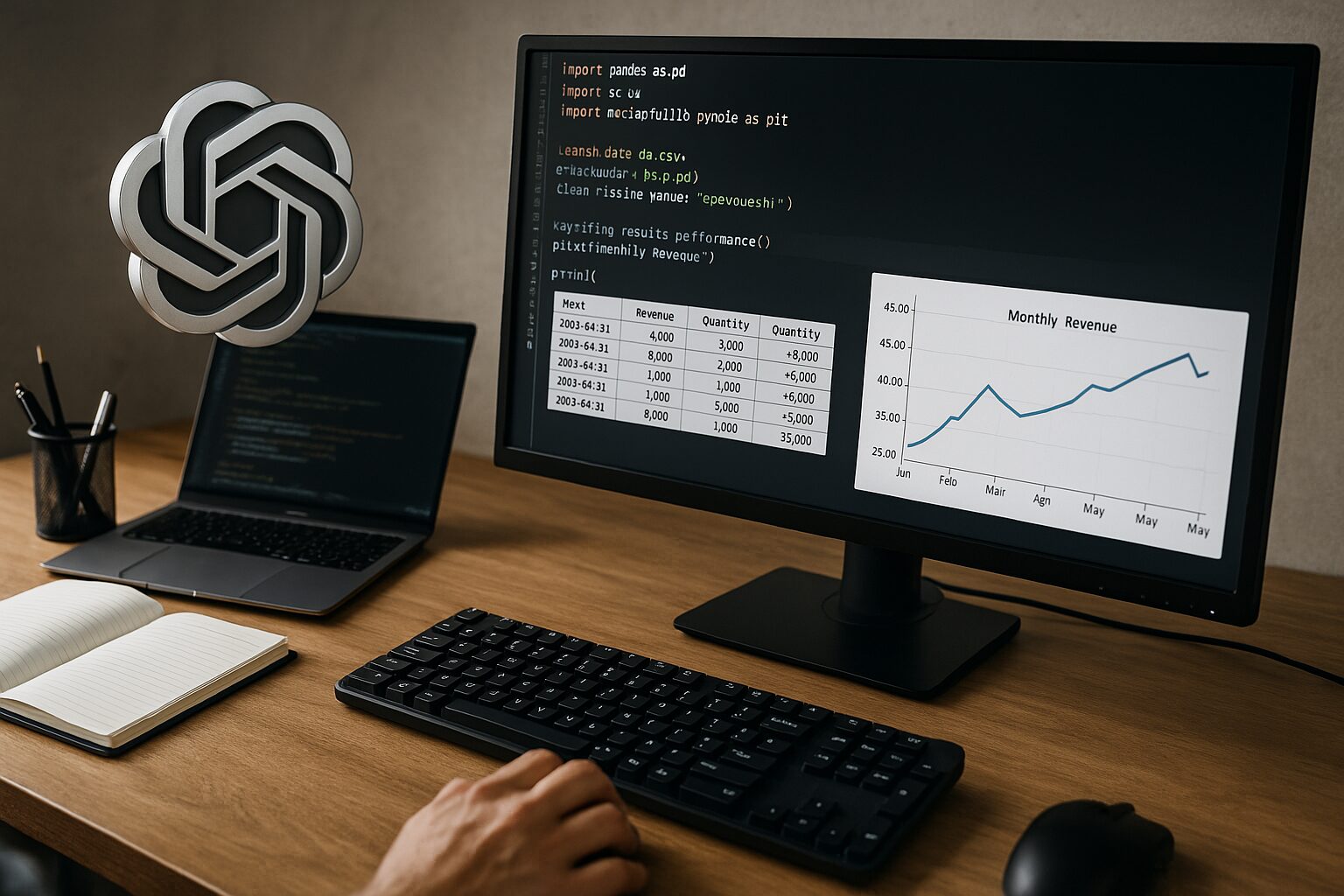
One of the key advantages of ChatGPT for data scientists is its ability to generate boilerplate code for tasks like data loading, cleaning, and visualization. Let’s explore how to effectively use ChatGPT for generating such scripts.
Crafting Effective Prompts
The quality of ChatGPT’s response largely depends on how well you frame your query. Here are a few tips for crafting prompts:
- Be Specific: Clearly describe what you want ChatGPT to do (e.g., “generate a Pandas script to load a CSV of user data and summarize it”).
- Provide Context: If you’re working with a particular data set or have specific columns, mention them.
- Set Constraints: If you want to avoid certain functions or use certain libraries, specify that.
An example prompt could be:
“Please write a Python script using Pandas to load a CSV file called
sales_data.csv
, clean any missing values, and generate summary statistics for the ‘Revenue’ and ‘Quantity’ columns.”
Data Wrangling with Pandas
Pandas is essential for any data scientist. With ChatGPT, you can quickly generate code for:
- Loading Data: Reading CSV, Excel, JSON, or SQL data.
- Cleaning and Transforming: Handling missing values, renaming columns, filtering rows, etc.
- Group By and Aggregations: Summarizing data by categories.
For instance, ChatGPT might output something like:
import pandas as pd
# Load the CSV file
df = pd.read_csv('sales_data.csv')
# Clean missing values
df = df.dropna()
# Generate summary statistics
revenue_summary = df['Revenue'].describe()
quantity_summary = df['Quantity'].describe()
print("Revenue Summary:")
print(revenue_summary)
print("\nQuantity Summary:")
print(quantity_summary)
Numerical Computations with NumPy
Although Pandas covers many data-manipulation needs, NumPy is indispensable for numerical computations like matrix operations, statistical functions, and advanced transformations. You can ask ChatGPT to help you:
- Perform array reshaping and manipulations.
- Implement custom mathematical formulas.
- Integrate NumPy arrays into Pandas workflows.
An example prompt:
“Show me how to use NumPy to create a 2D array of random numbers and compute the mean, median, and standard deviation along the columns.”
ChatGPT might suggest code like:
import numpy as np
# Create a 2D array of random numbers
arr = np.random.rand(5, 3) # 5 rows, 3 columns
# Compute statistics along the columns
mean_vals = np.mean(arr, axis=0)
median_vals = np.median(arr, axis=0)
std_vals = np.std(arr, axis=0)
print("Array:\n", arr)
print("\nMean values:", mean_vals)
print("Median values:", median_vals)
print("Standard Deviation values:", std_vals)
Plotting and Visualization with Matplotlib
Matplotlib is the go-to Python library for straightforward plots and charts. For more advanced visuals, libraries such as seaborn and plotly exist, but Matplotlib forms the foundation. You can ask ChatGPT to:
- Generate line plots, bar charts, histograms, scatter plots, etc.
- Customize axes, labels, and titles.
- Incorporate subplots for more complex visuals.
For example:
“Create a line plot of monthly revenue from a DataFrame using Matplotlib, setting the x-axis as the month column and the y-axis as total revenue.”
ChatGPT might respond with:
import matplotlib.pyplot as plt
# Assuming df has columns 'Month' and 'Revenue'
plt.plot(df['Month'], df['Revenue'])
plt.xlabel('Month')
plt.ylabel('Revenue')
plt.title('Monthly Revenue')
plt.show()
Example: A Simple Sales Analysis
Let’s say you have a dataset sales_data.csv
with columns Date
, Product
, Quantity
, and Revenue
. Here’s how a ChatGPT-generated script might look:
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# 1. Load the data
df = pd.read_csv('sales_data.csv')
# 2. Clean missing values
df.dropna(inplace=True)
# 3. Convert 'Date' column to datetime
df['Date'] = pd.to_datetime(df['Date'])
# 4. Group by month and summarize
df['Month'] = df['Date'].dt.to_period('M')
monthly_revenue = df.groupby('Month')['Revenue'].sum().reset_index()
# 5. Plot monthly revenue
plt.plot(monthly_revenue['Month'].astype(str), monthly_revenue['Revenue'])
plt.xlabel('Month')
plt.ylabel('Total Revenue')
plt.title('Monthly Revenue Trend')
plt.xticks(rotation=45)
plt.tight_layout()
plt.show()
# 6. Basic statistics
print("Basic Statistics on Revenue:")
print(df['Revenue'].describe())
With this straightforward example, ChatGPT has done a large chunk of the boilerplate coding, letting you focus on interpretation and insights.
Building Machine Learning Pipelines
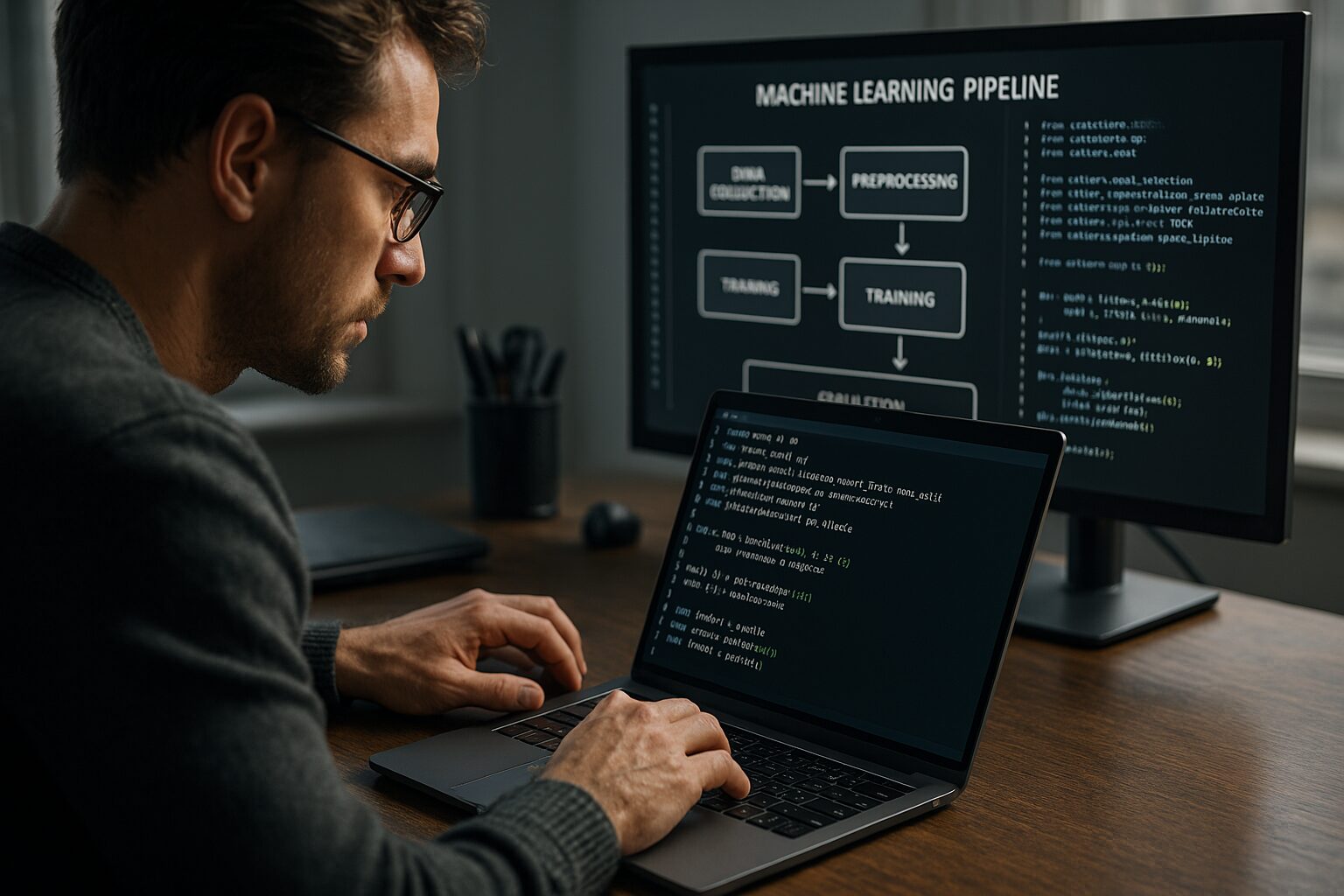
Moving beyond data analysis, ChatGPT can also help you craft complete machine learning pipelines. The following sections detail how to integrate ChatGPT’s coding assistance into your ML workflows.
Overview of ML Pipelines
In scikit-learn, an ML pipeline typically involves:
- Data Preprocessing: Handling missing values, encoding categorical variables, scaling numerical features.
- Model Selection: Choosing an appropriate algorithm (e.g., Logistic Regression, Random Forest, etc.).
- Training and Evaluation: Splitting data into training and testing sets, fitting the model, and evaluating performance metrics.
- Hyperparameter Tuning: Optimizing model parameters (e.g., using GridSearchCV).
ChatGPT-Suggested Models and Use Cases
ChatGPT can suggest models based on the type of problem you describe:
- Classification: Logistic Regression, Decision Trees, Random Forests, XGBoost, etc.
- Regression: Linear Regression, Ridge/Lasso, Random Forest Regressor, etc.
- Clustering: K-Means, DBSCAN, Hierarchical Clustering.
- Time Series: ARIMA, Prophet (though not a part of scikit-learn, but can be suggested for time-series data).
For instance, if you have a binary classification problem (predicting whether a customer will churn or not), ChatGPT might recommend using LogisticRegression
or a RandomForestClassifier
.
Implementing scikit-learn Templates
A typical scikit-learn classification pipeline might look like this:
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score, classification_report
from sklearn.pipeline import Pipeline
# Example DataFrame: df with features in X and target in y
X = df.drop('target', axis=1)
y = df['target']
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y,
test_size=0.2,
random_state=42)
# Define a pipeline
pipeline = Pipeline([
('scaler', StandardScaler()),
('clf', LogisticRegression())
])
# Train pipeline
pipeline.fit(X_train, y_train)
# Predictions
y_pred = pipeline.predict(X_test)
# Evaluate
print("Accuracy:", accuracy_score(y_test, y_pred))
print(classification_report(y_test, y_pred))
Example: Classification Pipeline
Let’s say you have a dataset for a bank’s marketing campaign, where you want to predict whether a client will subscribe to a new product (subscribe
= yes/no). An example ChatGPT prompt could be:
“Write a classification pipeline in scikit-learn to predict if customers will subscribe to a new product, using a logistic regression model with scaling.”
You might get a detailed pipeline like the snippet above. ChatGPT might also suggest parameter tuning strategies or alternative models like Random Forest for better performance.
Interpreting Results and Explaining Outcomes
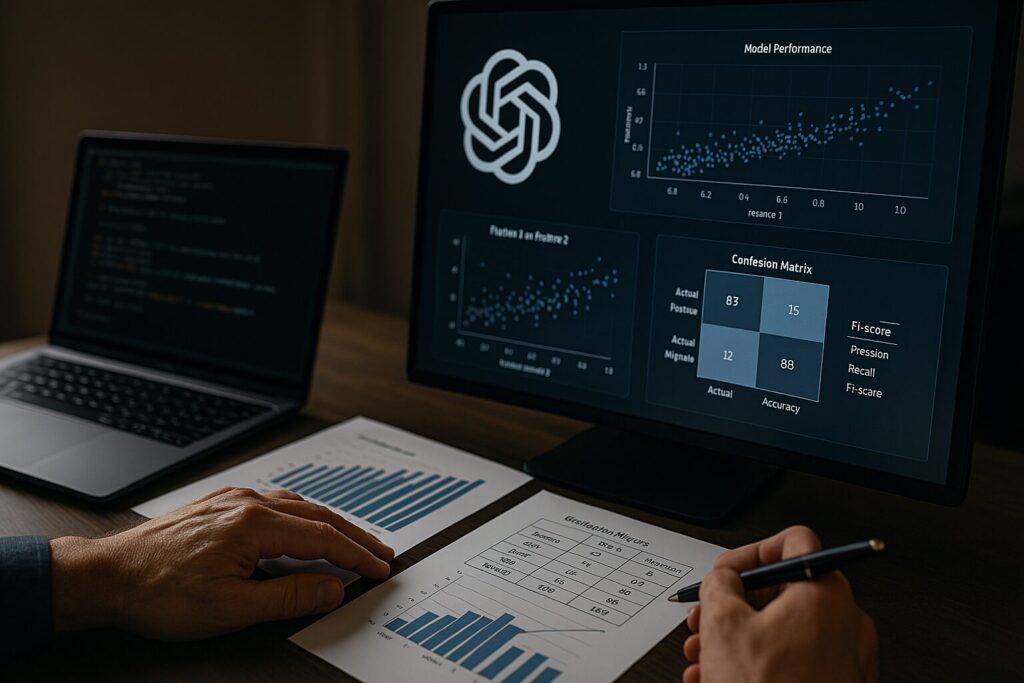
ML models and data analysis are only as good as your ability to interpret the results. ChatGPT can help you summarize outcomes, explain metrics, and provide insights into how a model arrived at certain conclusions.
Summarizing Statistical Findings
When you run statistical analyses (t-tests, ANOVA, correlations, etc.), the raw output can be cryptic. ChatGPT can:
- Convert raw statistical output into layman’s terms.
- Highlight important p-values, effect sizes, or correlations.
- Suggest potential follow-up analyses.
For instance, if you run a t-test in Python:
from scipy.stats import ttest_ind
stat, p_value = ttest_ind(group1, group2)
You can paste the stat
and p_value
into ChatGPT along with any context, and ask:
“Please interpret these t-test results. What do they mean for the difference between the two groups?”
ChatGPT might respond with a summary explaining whether the difference is statistically significant and the implications for your hypothesis.
Explaining ML Model Outputs
Confusion matrices, classification reports, and regression coefficients can be confusing to less-experienced practitioners. ChatGPT can help you break down these outputs:
- Confusion Matrix: Explains true positives, false positives, true negatives, and false negatives.
- Classification Report: Discusses precision, recall, f1-score, and support.
- Regression Coefficients: Interprets slope (beta) coefficients, p-values, and confidence intervals.
How ChatGPT Helps in Model Interpretation
While ChatGPT does not have direct access to your model internals, you can provide:
- Coefficients or Feature Importances: Copy-paste them into ChatGPT.
- Performance Metrics: E.g., R^2, accuracy, F1-score, confusion matrix results.
ChatGPT will then create a narrative describing how each feature impacts the model, which metrics are strong or weak, and what you might do to improve it.
Example: Explaining Regression Outputs
Consider a linear regression scenario predicting house prices:
from sklearn.linear_model import LinearRegression
model = LinearRegression()
model.fit(X_train, y_train)
coefficients = model.coef_
intercept = model.intercept_
r2_score = model.score(X_test, y_test)
You can then provide ChatGPT with:
- The feature names (
X_train.columns.tolist()
) - The
coefficients
array - The
intercept
- The
r2_score
Ask ChatGPT:
“Explain what these coefficients mean and whether the R^2 score of 0.78 indicates a good model.”
ChatGPT might respond by telling you how each coefficient correlates with price, the meaning of the intercept, and what an R^2 of 0.78 indicates in terms of the variance explained in the data.
Prompting ChatGPT for Optimization
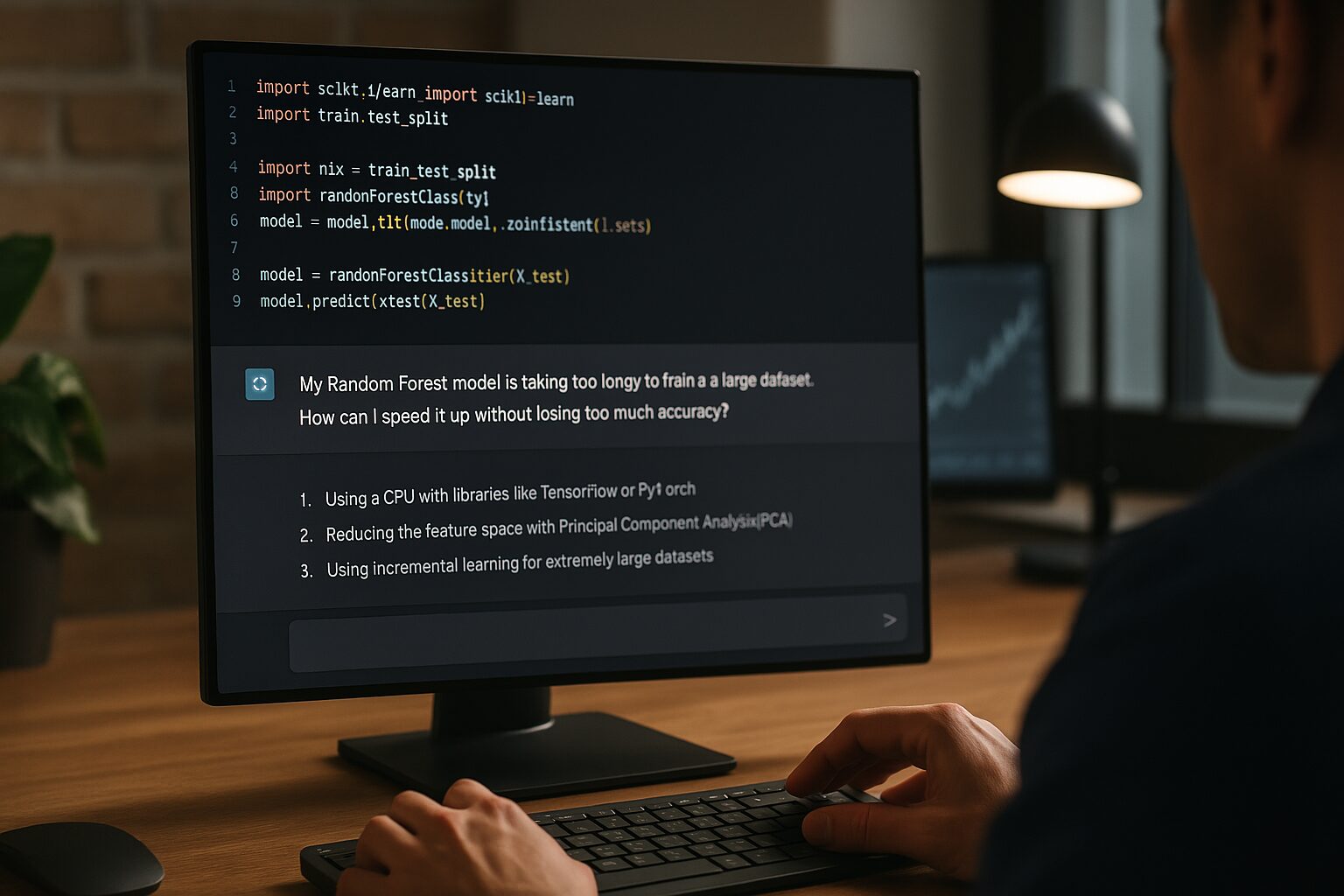
Beyond generating scripts, ChatGPT can serve as a guide for optimizing both data analysis workflows and machine learning models. This includes performance optimization, code refactoring, and hyperparameter tuning.
Performance Tuning in Data Analysis Scripts
Large data sets can lead to slow Pandas operations. ChatGPT can help:
- Vectorization: Replacing loops with vectorized operations.
- Batch Processing: Loading data in chunks for memory efficiency.
- Parallelization: Suggesting libraries like
Dask
or joblib to parallelize tasks.
For example, if you’re merging multiple DataFrames or performing nested loops, you can ask ChatGPT:
“How can I improve this merge operation to handle 10 million rows efficiently?”
ChatGPT might suggest using efficient join operations, indexing, or chunk-based processing.
Optimizing ML Models
For ML pipelines, optimization might involve:
- Algorithm Choice: Trying different algorithms (e.g., from linear models to tree-based methods).
- Hyperparameter Tuning: Using grid search, random search, or Bayesian optimization to find the best parameters.
- Feature Engineering: Reducing dimensionality or creating new features to improve model performance.
Hyperparameter Tuning with ChatGPT
You can ask ChatGPT for code examples to use GridSearchCV
or RandomizedSearchCV
in scikit-learn. For instance:
“Generate code to perform GridSearchCV on a RandomForestClassifier with parameters for n_estimators and max_depth.”
ChatGPT could generate:
from sklearn.model_selection import GridSearchCV
from sklearn.ensemble import RandomForestClassifier
params = {
'n_estimators': [50, 100, 150],
'max_depth': [None, 10, 20]
}
grid_search = GridSearchCV(RandomForestClassifier(), params, cv=5)
grid_search.fit(X_train, y_train)
print("Best parameters:", grid_search.best_params_)
print("Best score:", grid_search.best_score_)
Example: Improving Training Efficiency
If your training is running too slowly, ChatGPT might suggest:
- Using a GPU with libraries like TensorFlow or PyTorch (if neural networks are involved).
- Reducing Feature Space with Principal Component Analysis (PCA).
- Using Incremental Learning for extremely large datasets.
You could prompt:
“My RandomForest model is taking too long to train on 2 million rows of data. How can I speed this up without losing too much accuracy?”
ChatGPT might respond by suggesting:
- Downsizing data or using partial fit if feasible.
- Feature selection or dimensionality reduction.
- Tuning parallel processing parameters (
n_jobs
).
Why This Matters & Future Outlook
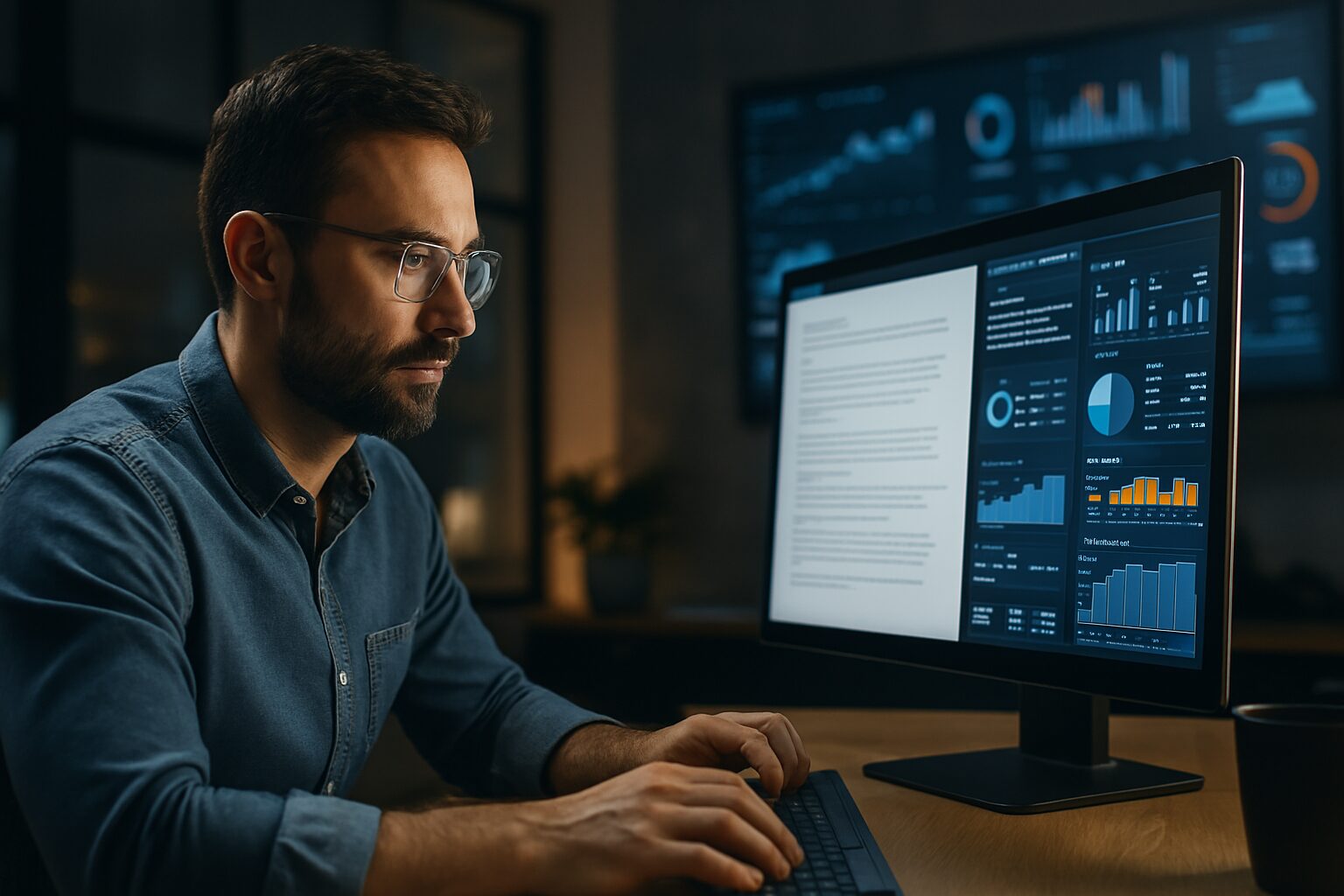
The Growing Importance of AI in Data Science
AI tools like ChatGPT are not just novelties; they’re transformative. As data grows in volume and complexity, AI-driven assistants become vital in:
- Reducing the Skill Gap: Less experienced data analysts can produce robust work with AI guidance.
- Saving Time: Repetitive tasks become quicker, letting you focus on higher-level strategy.
- Enhancing Collaboration: ChatGPT can mediate between business stakeholders and data science teams by summarizing technical details in an accessible format.
Practical Impact on Productivity
- Rapid Prototyping: Quickly draft scripts for EDA, freeing you to focus on strategic questions.
- Better Code Quality: AI suggestions often incorporate best coding practices, making your code more readable and efficient.
- Instant Feedback: ChatGPT can act as a second pair of eyes, providing real-time code reviews.
Challenges and Ethical Considerations
- Data Privacy: Be cautious about sharing sensitive data with ChatGPT or any AI system. Ensure anonymization or summarization where possible.
- Model Bias: AI-suggested code or analysis might inadvertently perpetuate biases. Always review your data sources and results carefully.
- Reliance on AI: Over-reliance on ChatGPT may hamper your own coding skills. Strike a balance between AI assistance and manual practice.
Long-Term Outlook
Tools like ChatGPT will evolve to offer more specialized and robust data science features, potentially integrating with major IDEs and data platforms. Expect more advanced debugging features, real-time code suggestions, and deeper integration with cloud-based data environments. The synergy between AI and data science is only just beginning.
Final Thoughts
ChatGPT is a versatile assistant for data analysis and machine learning in Python, offering support throughout the entire process—from data cleaning and visualization to building and optimizing ML pipelines. By writing effective prompts, you can leverage ChatGPT to accelerate your workflow, reduce coding errors, and discover new approaches you might otherwise overlook.
While AI-powered tools can drastically cut down on development time, they are not a substitute for understanding the underlying concepts of data science and machine learning. Always review the code, validate your methods, and ensure ethical practices. As you become more comfortable with AI-assisted coding, you’ll find a balance between manual expertise and AI guidance—unlocking unprecedented productivity and insight.
Summary of Key Takeaways
- Data Analysis Made Easy: Use ChatGPT to quickly generate scripts for data loading, cleaning, summarization, and visualization.
- Seamless ML Pipelines: Get end-to-end machine learning pipelines (preprocessing, model selection, evaluation) drafted in seconds.
- Interpretation & Explanation: ChatGPT can translate raw statistical or ML model outputs into clear, actionable insights.
- Optimization Guidance: From vectorizing Pandas operations to hyperparameter tuning, ChatGPT helps you identify performance bottlenecks.
- Future Impact: AI-driven development is here to stay, offering an ever-growing suite of possibilities for data professionals.
By implementing these best practices and harnessing the power of ChatGPT, you’ll be well on your way to accelerating your Python-based data analysis and machine learning projects—allowing you to spend more time drawing meaningful conclusions and less time wrestling with boilerplate code or repetitive tasks.