In recent years, Python has emerged as one of the most popular programming languages, known for its readability, robust ecosystem, and versatility. Meanwhile, ChatGPT—an advanced language model developed by OpenAI—has captured the imagination of developers for its ability to generate text, draft content, and even write or debug code on demand.
This article offers a detailed, step-by-step roadmap to get you started with using ChatGPT to generate Python code. Whether you’re a novice just learning your first print()
statement or a seasoned developer looking to speed up your workflow, ChatGPT can be an invaluable companion.
We’ll explore everything from setting up your environment to crafting effective prompts to debugging and refining the code ChatGPT provides. By the end, you’ll not only understand how to integrate ChatGPT into your Python projects but also gain insights into best practices, ethical considerations, and common pitfalls.
Table of Contents
What is ChatGPT and Why Does It Matter for Python Developers?
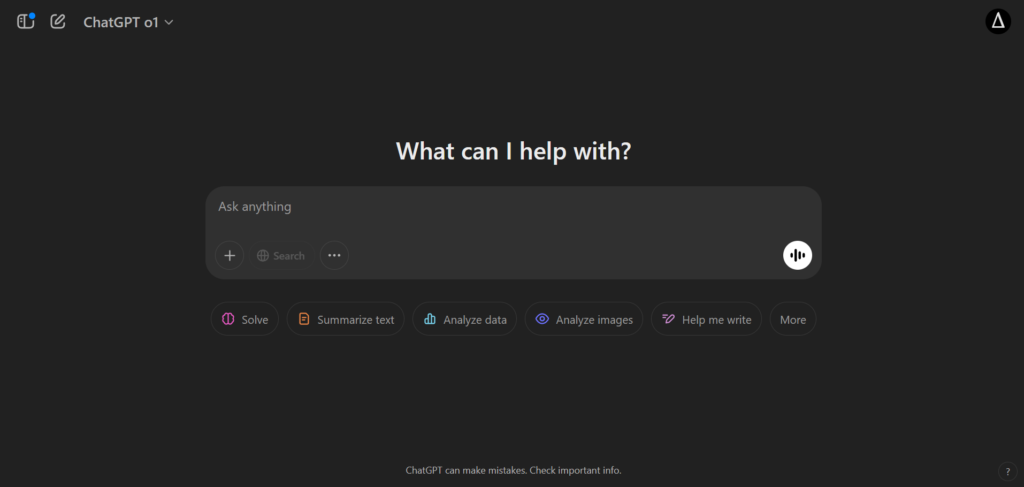
ChatGPT is a specialized instance of the GPT (Generative Pre-trained Transformer) architecture, fine-tuned for interactive conversations and a wide array of tasks, including code generation. By harnessing a huge corpus of text, ChatGPT can interpret prompts in plain English (or other languages) and respond with detailed, context-specific answers.
Why ChatGPT is Relevant
- Accessibility: You don’t need a background in machine learning to start using ChatGPT. It’s designed for natural language interactions.
- Time-Efficiency: By generating boilerplate code, performing initial debugging, or providing code outlines, ChatGPT can significantly reduce development time.
- Versatility: From web development frameworks like Flask and Django to data science libraries like NumPy or Pandas, ChatGPT can assist with a wide range of Python libraries and use cases.
Impact on Developer Workflows
Traditionally, developers cycle through documentation, Q&A platforms, and trial-and-error to solve coding challenges. While these methods remain valuable, ChatGPT offers a single interface that can speed up the entire process—from conceptualizing code to testing small snippets—acting as a dynamic ally in your coding journey.
Setting Up Your Environment
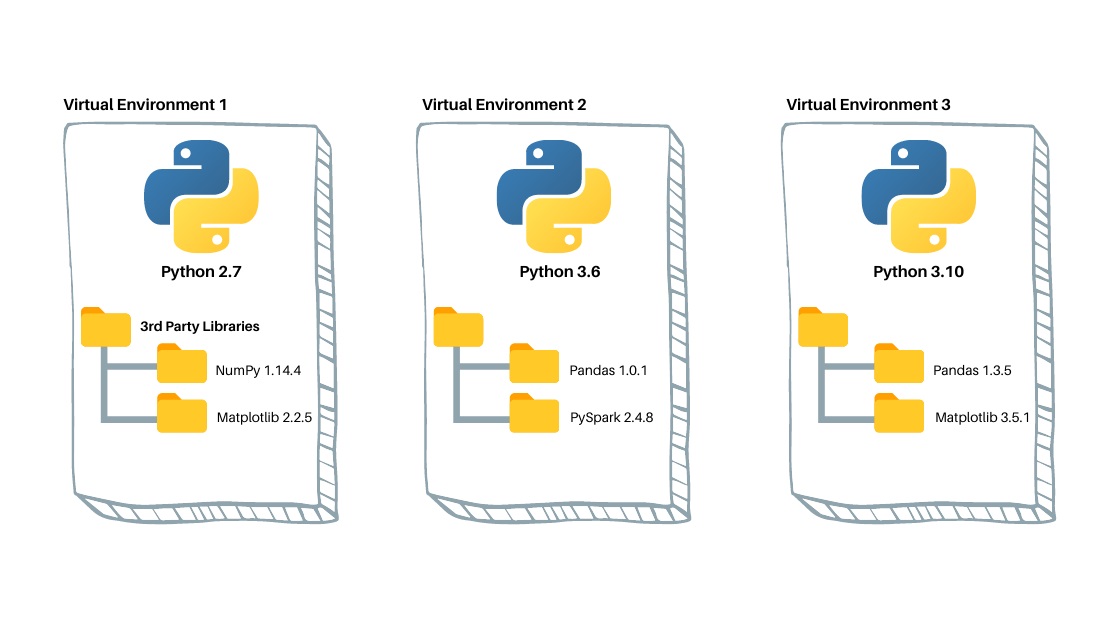
Before you can start using ChatGPT to generate Python code, it’s essential to have a proper development environment. This section outlines the necessary tools and configurations.
1. Install Python
- Latest Python Version: Download from python.org. The recommended versions at the time of writing are Python 3.9+ or Python 3.10+.
- Check Installation: Open a terminal and run:
python --version
If it returnsPython 3.x.x
, you’re good to go.
2. Create a Virtual Environment
Using a virtual environment isolates your project’s dependencies:
python -m venv venv
source venv/bin/activate # For macOS/Linux
venv\Scripts\activate # For Windows
3. Install Libraries
Depending on what type of code ChatGPT generates, you may need certain libraries. A few common ones include:
pip install requests pandas numpy
While you can always install on-demand, it helps to have common data manipulation and networking libraries at the ready.
4. Access ChatGPT
- Web Interface: The simplest way is to use chat.openai.com.
- OpenAI API: For more advanced tasks, you can sign up for the OpenAI developer platform and obtain an API key. You can then use the
openai
Python library to interact with ChatGPT programmatically.
Key Benefits of Generating Python Code with ChatGPT
Harnessing ChatGPT for Python code brings several distinct advantages:
- Speed: Generating boilerplate code or standard functions (like connecting to an API) can be done almost instantly.
- Learning Resource: Beginners can glean insights into proper syntax and structures by observing ChatGPT’s output.
- Reduced Errors: While it’s not foolproof, ChatGPT often handles the smaller details of code that are easy to forget or overlook.
- Rapid Prototyping: Quickly spin up new features, test scripts, or entire project components with minimal effort.
How ChatGPT Works: A Closer Look
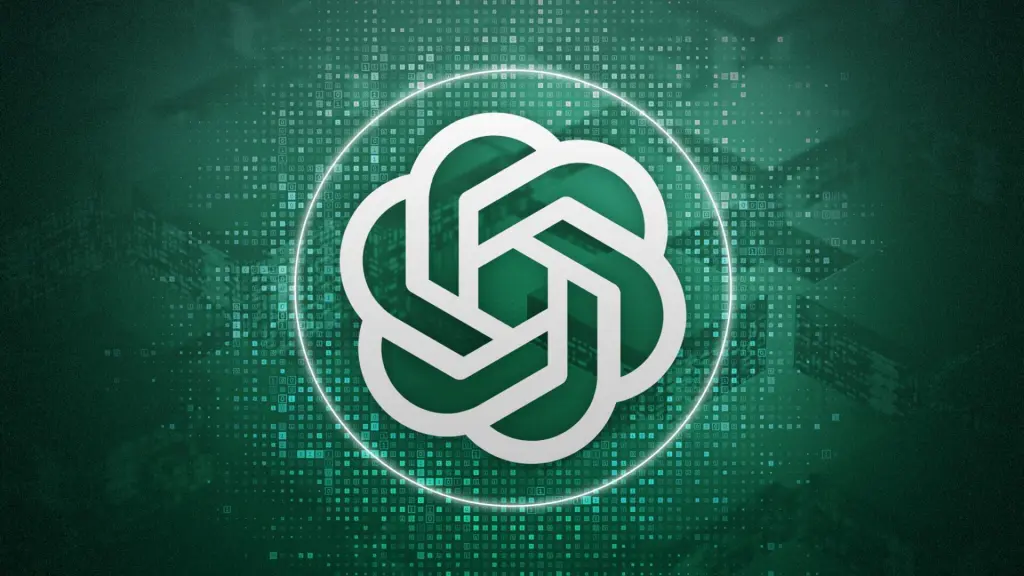
To truly leverage ChatGPT’s capabilities for Python code generation, it helps to understand its underlying mechanics.
The GPT Family
ChatGPT belongs to the GPT (Generative Pre-trained Transformer) family, which uses the Transformer neural network architecture. The model is pre-trained on vast datasets, including websites, books, and forums, giving it a broad textual understanding.
Fine-Tuning for Code
OpenAI has introduced specialized fine-tuning (e.g., models like GPT-3.5, GPT-4, or Codex) that enhance the model’s ability to deal with programming languages. Through this, ChatGPT becomes adept at:
- Writing Pythonic code.
- Suggesting library-specific functionalities.
- Parsing error messages and offering debugging tips.
Conversation and Context
ChatGPT keeps a context window of the conversation. This means it can remember previous messages (up to a certain token limit), allowing for iterative questioning and refinement of code. However, long or complex dialogues can exceed this limit, which may cause the model to lose some context.
Basics of Prompting ChatGPT for Python Code
When it comes to generating Python code, the quality of your prompt is paramount. Consider the following elements:
- Task Clarification: Clearly state what you want ChatGPT to do (e.g., “Generate a function to parse JSON.”).
- Environment Details: Specify any library versions, environment constraints, or coding conventions.
- Expected Format: Clarify if you want a code snippet, a function, a class, or an entire script.
Crafting Your First Prompt
For instance, if you want to fetch and analyze data from a public API, you could say:
“Create a Python script that uses the
requests
library to fetch JSON data fromhttps://api.example.com/data
, parse the result, and print a summary of the keys.”
Why This Works
- Clarity: You stated exactly which library to use.
- Specificity: You provided a target URL and the task (parse JSON, print a summary).
- Expected Outcome: You asked for a script, so ChatGPT will likely include an executable
if __name__ == "__main__":
block or a main function.
Prompt Engineering: Getting the Best Out of ChatGPT
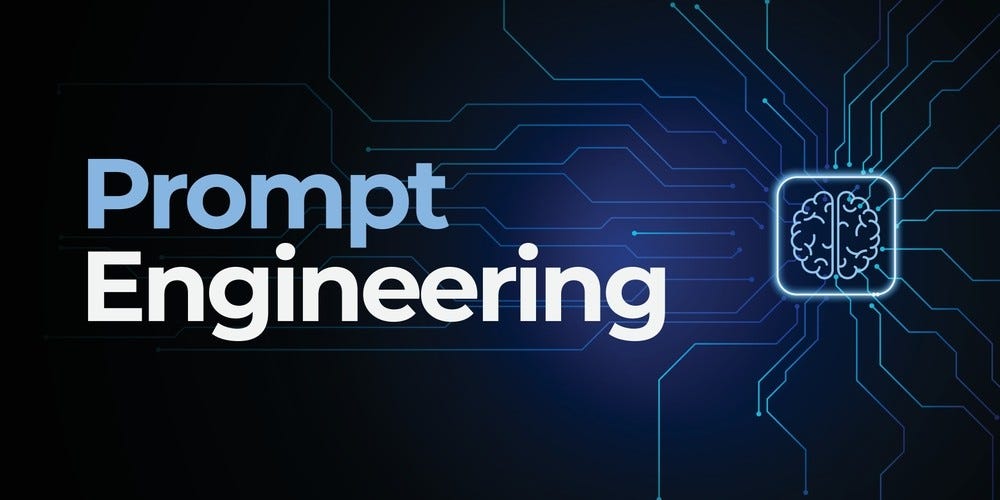
Prompt engineering is the art (and science) of constructing prompts that maximize the usefulness and accuracy of ChatGPT’s outputs.
Strategies for Better Prompts
- Contextual Depth: Provide background information. For example, “In a Django project, define a model…”
- Constraints: If you have memory or performance constraints, mention them.
- Request Explanations: Ask ChatGPT to include comments or docstrings to understand the code it generates.
Example
“Generate a Python function using Pandas to read a CSV of financial transactions, grouped by ‘AccountID,’ and calculate the total sum of ‘Amount.’ Include docstrings and inline comments explaining each step.”
Iterative Refinement
- Follow-Up Queries: If ChatGPT doesn’t cover every detail, keep refining your prompt: “Can you modify the code to handle missing ‘Amount’ values by treating them as zeros?”
- Multiple Versions: You can also request alternative solutions: “Please provide both an iterative approach and a vectorized approach for performance comparison.”
Step-by-Step Example: Generating a Simple Python Script with ChatGPT
Let’s walk through a concrete scenario of using ChatGPT to generate a basic script.
1. Scenario
We want a script that:
- Reads a list of product details from a CSV file (columns:
product_id
,product_name
,price
). - Calculates the total number of products and the average price.
- Prints the output to the console.
2. Drafting the Prompt
“Generate a Python script that reads a CSV file named ‘products.csv’ with columns ‘product_id’, ‘product_name’, and ‘price’. Use pandas to compute the total number of products and the average price, then print these values. Include docstrings and inline comments.”
3. Potential ChatGPT Output
import pandas as pd
def analyze_products(csv_file: str):
"""
Reads a CSV file containing product details and computes:
1. The total number of products
2. The average product price
:param csv_file: Path to the products CSV file
:return: A tuple (total_products, average_price)
"""
# Read the CSV file into a pandas DataFrame
df = pd.read_csv(csv_file)
# Calculate the total number of products
total_products = df.shape[0]
# Convert price column to numeric in case of data issues
df['price'] = pd.to_numeric(df['price'], errors='coerce')
# Calculate the average price, ignoring NaN values
average_price = df['price'].mean()
return total_products, average_price
if __name__ == "__main__":
file_path = "products.csv"
# Call our function to analyze the products
total, avg = analyze_products(file_path)
# Print the results
print(f"Total number of products: {total}")
print(f"Average product price: {avg:.2f}")
4. Analyzing the Output
- Docstrings: Present in the main function (
analyze_products
). - Inline Comments: Explain each step, from reading the CSV to calculating the average.
- Type Hints: Python 3 type hints (
str
) show a more modern coding style.
This code example is typically well-structured, but you’d still need to verify it with real data and ensure that your CSV file matches the expected columns.
Validating and Refining Generated Code
Even high-quality code from ChatGPT requires validation and, sometimes, refinement. Always run the generated code in your IDE or terminal to confirm it works as intended.
Steps to Validate
- Run a Test: If you have a CSV file, ensure it’s in the correct format and run the script.
- Inspect Edge Cases: What happens if the CSV file is empty? Contains invalid data?
- Add Logging or Prints: Insert log statements or print statements to observe data at key checkpoints.
Continuous Feedback Loop
If the code isn’t perfect, copy your error messages or concerns back into ChatGPT:
“I received a ValueError: ‘could not convert string to float.’ How can I handle invalid price entries gracefully?”
This iterative process helps refine the solution further.
Iterative Development and Debugging with ChatGPT
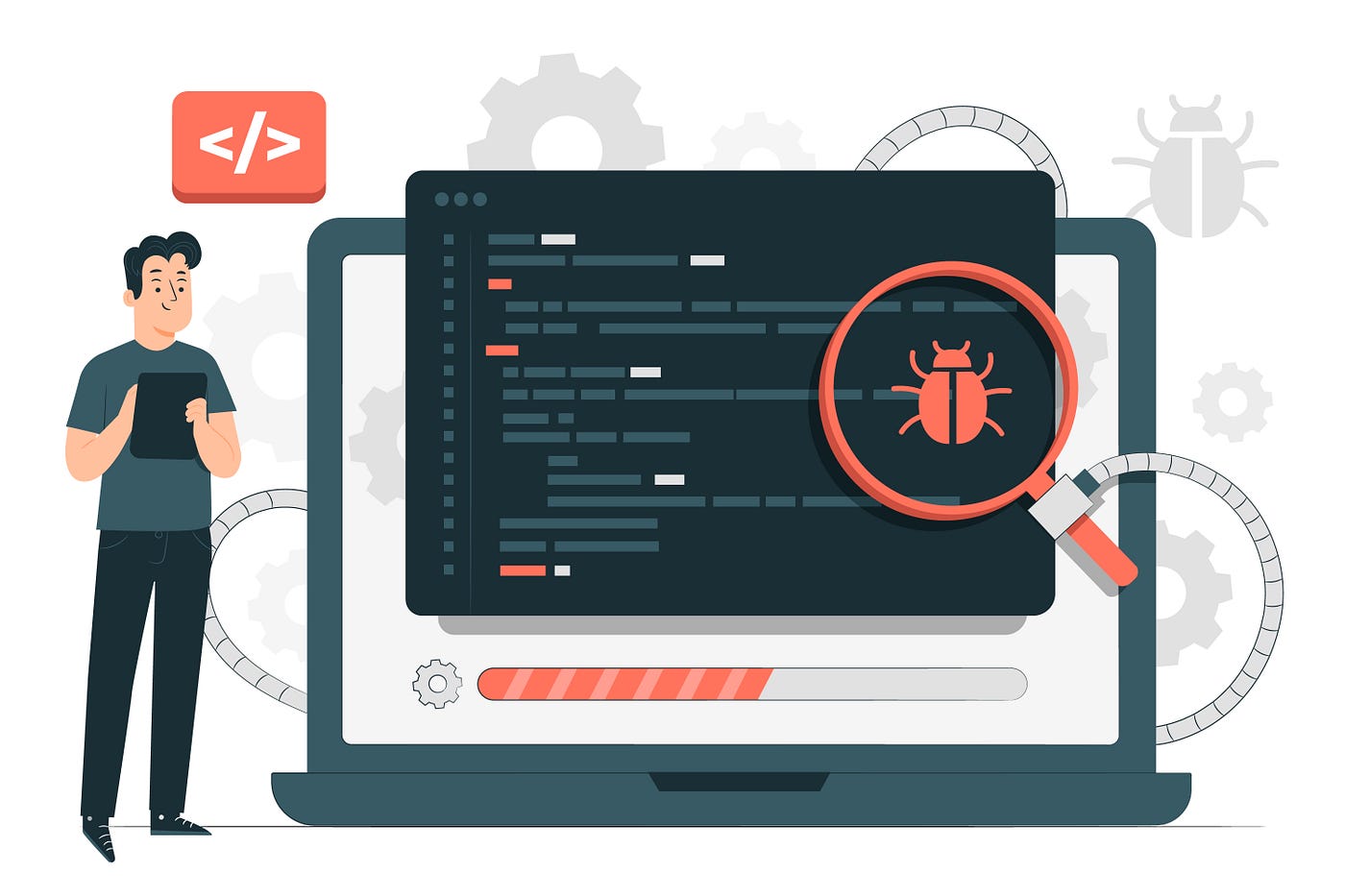
In real-world applications, you rarely get everything right on the first try. ChatGPT excels in an iterative approach to development.
Debugging
- Provide Error Output: Paste the full traceback or error message into ChatGPT.
- Explain Context: Mention library versions or relevant environment details.
- Ask for Step-by-Step Guidance: Encourage ChatGPT to detail its reasoning or propose multiple fixes.
Example Prompt
“I tried the previous code, but I’m getting a KeyError: ‘price’ in the DataFrame. Could you help me debug and suggest how to handle missing columns?”
Enhancement Cycles
Even after the code works, you may want to optimize or add features. For example:
- More columns: If your CSV includes a ‘category’ column, ask ChatGPT to group by category and calculate metrics.
- Efficiency: If your dataset is huge, prompt ChatGPT for chunked data loading using
pandas.read_csv(..., chunksize=10000)
.
Advanced Use Cases and Techniques
As you grow more comfortable with ChatGPT, you can move beyond basic generation into advanced techniques.
Code Refactoring
Prompt ChatGPT to:
- Enforce PEP 8 or your team’s coding style.
- Convert lengthy scripts into class-based designs or use design patterns.
- Improve performance by vectorizing loops, implementing caching, or migrating to asynchronous I/O.
Integration with Frameworks
- Django: Have ChatGPT auto-generate models, views, or URL configurations.
- Flask: Ask for boilerplate app code, request handling, or database integration.
- FastAPI: Generate endpoint functions with pydantic models for data validation.
Automated Documentation
Request ChatGPT to generate docstrings or README sections explaining how the code works. This can be a boon for open-source contributions or team-based projects where clarity is vital.
Best Practices for Security, Licensing, and Ethical Use
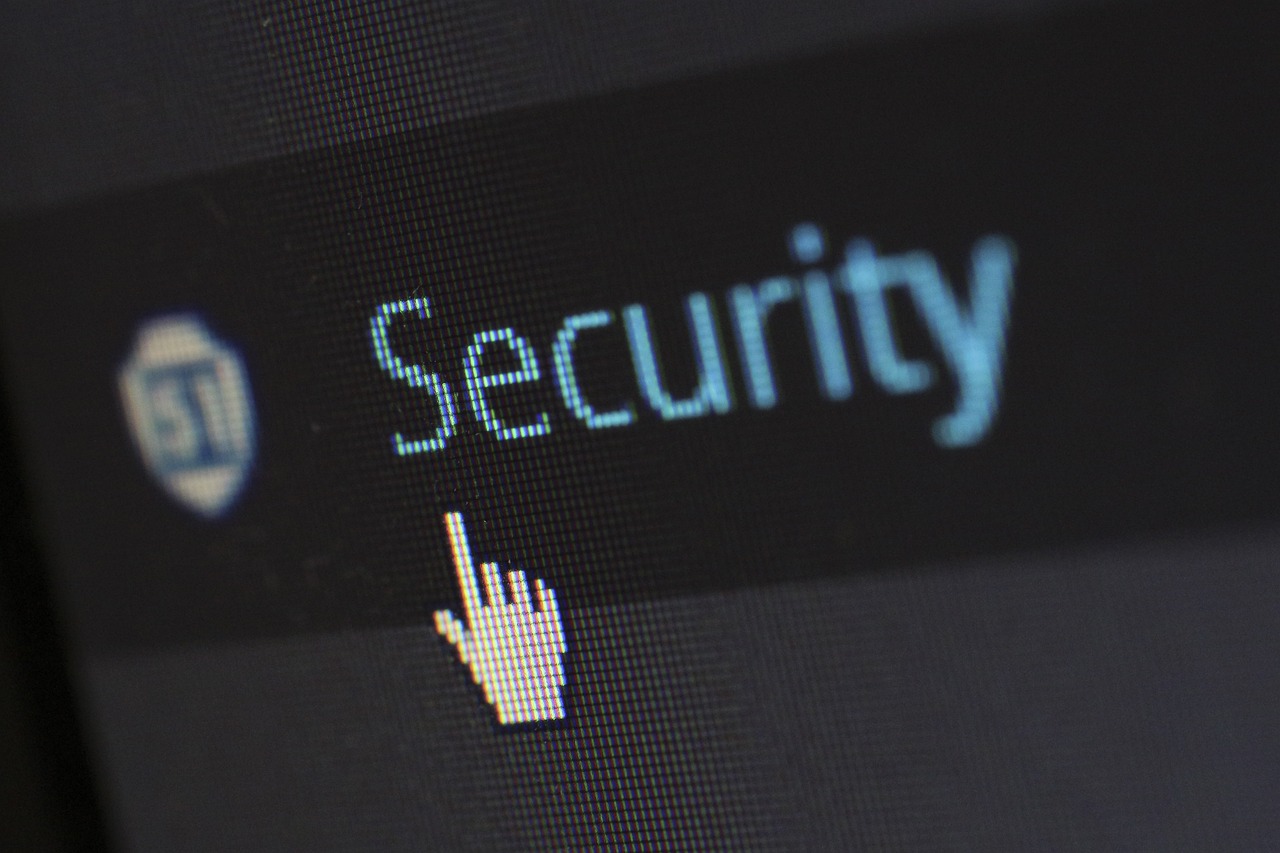
While ChatGPT is powerful, you should maintain a careful approach regarding security, licensing, and ethics.
Security
- Secret Keys: Never paste sensitive keys or passwords into a prompt. ChatGPT data may be logged or used for model improvements, so keep sensitive info private.
- SQL Injection and Vulnerabilities: Review any code that handles user input, especially if ChatGPT suggests direct database queries.
Licensing Concerns
- OpenAI Terms: Check OpenAI’s policy and usage terms, particularly if you plan to commercialize or widely distribute your AI-assisted code.
- Code Attribution: If your organization requires it, keep track of which parts were AI-generated for legal or compliance reasons.
Ethical Use
- Bias and Misinformation: ChatGPT may reflect biases from its training data. If it suggests problematic or incomplete logic, apply human oversight.
- Intellectual Property: Ensure you don’t inadvertently replicate proprietary code or violate licenses through AI-generated snippets.
Common Pitfalls and How to Avoid Them
Even the most advanced tool has limitations. By anticipating potential pitfalls, you can preempt issues.
- Hallucinated Code: ChatGPT might produce code for libraries that don’t exist or functions that aren’t real. Double-check functionality in official documentation.
- Incomplete Context: If your conversation is extensive, ChatGPT may lose earlier context. Split the conversation or re-summarize your context.
- Version Conflicts: ChatGPT might assume you’re using the latest versions of libraries. If you’re on older versions, specify in your prompt.
- Performance Oversights: Generated code may not consider memory usage or time complexity. Always test with real data scales.
Practical Applications and Real-World Scenarios
Data Processing Scripts
Small businesses or data enthusiasts can quickly generate scripts to automate tasks:
- Merging CSV Files: ChatGPT can outline a quick approach to read multiple CSV files, combine them, and create a unified dataset.
- Data Cleaning: Get suggestions on how to handle missing values, outliers, or data validation steps.
Web Scraping
Python is popular for web scraping tasks using libraries like requests
or BeautifulSoup
. ChatGPT can:
- Suggest boilerplate for making HTTP requests, parsing HTML, and storing results in a CSV.
- Provide tips for rotating proxies or managing concurrency with
asyncio
.
API Integrations
If you want to integrate with third-party APIs, ChatGPT can:
- Generate the authentication flow (e.g., OAuth).
- Outline how to handle pagination, rate limits, and error responses.
Machine Learning Prototypes
Data scientists or enthusiasts can use ChatGPT to:
- Generate data preprocessing code for scikit-learn or TensorFlow.
- Outline a simple classification or regression pipeline, with placeholders for hyperparameter tuning or validation splits.
Frequently Asked Questions (FAQ)
Q1. Is ChatGPT-generated code always correct?
Answer: Not necessarily. ChatGPT can produce syntactically correct but logically flawed code. Always test and review outputs thoroughly.
Q2. Can ChatGPT handle large, multi-file Python projects?
Answer: ChatGPT can generate modules, classes, or file structures, but it’s best at smaller chunks of code. For complex architectures, break down requests into manageable prompts and combine them manually.
Q3. Does ChatGPT replace the need to learn Python?
Answer: No. ChatGPT is a tool, not a substitute for understanding programming fundamentals. You still need foundational Python skills to interpret, validate, and maintain the code.
Q4. How do I handle confidential or proprietary logic?
Answer: Avoid directly pasting sensitive data. Summarize issues or rename variables to maintain confidentiality.
Q5. Can I integrate ChatGPT into my IDE?
Answer: While official ChatGPT plugins might exist for some IDEs, you often need a third-party extension or you can integrate the OpenAI API yourself for a custom in-IDE experience.
Conclusion
Getting Started: Using ChatGPT to Generate Python Code marks a paradigm shift in how developers approach coding tasks—whether they’re dealing with simple data parsing scripts or complex machine learning projects. By fusing Python’s flexibility and ChatGPT’s ability to produce (and debug) code quickly, programmers can:
- Accelerate project timelines by offloading boilerplate generation and initial testing.
- Improve the learning curve for newbies by offering real-time feedback and examples.
- Enhance creativity and experimentation through easy iterative refinements.
Key Takeaways
- Prompt Engineering: Effective prompts are the bedrock of accurate code generation.
- Validation: Always run tests and check the logic behind AI-generated suggestions.
- Iterative Development: ChatGPT shines when you refine your requests and incorporate feedback loops.
- Ethical and Secure Usage: Keep licensing and confidentiality in mind.
- Future Outlook: As models evolve, expect improved context handling, domain-specific fine-tuning, and deeper IDE integrations—further blurring the line between AI suggestions and human-driven coding.
By adopting these best practices, you ensure that ChatGPT becomes a valuable ally rather than just a novelty. Whether you’re crafting your first Python script or expanding a sophisticated application, ChatGPT holds the potential to streamline your development process, inspire solutions, and ultimately help you achieve your coding goals faster and more efficiently.